문제 : 거스름돈을 최소 개수로 되돌려 줄 때 필요한 거스름돈 수
거스름돈은 5만원부터 10원까지 있음.
제약 사항 : 입력하는 돈 N은 10~1,000,000이하의 정수, N의 마지막 자릿수는 항상 0
코드
#include <stdio.h>
#include <stdlib.h>
int main(void){
int i, j, n, testCase;
int money [8] = {50000, 10000, 5000, 1000, 500, 100, 50, 10};
int **answer;
scanf("%d", &testCase);
answer = (int**)malloc(sizeof(int*)*testCase);
for(i=0; i<testCase; i++) answer[i] = (int*)malloc(sizeof(int)*8);
for(i=0; i<testCase; i++){
scanf("%d", &n);
for(j=0; j<8; j++){
answer[i][j] = n/money[j];
n%=money[j];
}
}
for(i=0; i<testCase; i++){
printf("#%d\n", i+1);
for(j=0; j<8; j++){
printf("%d ", answer[i][j]);
}
printf("\n");
}
return 0;
}
이전과 풀었을 때와 달리 배열에 거스름돈 종류를 넣으면서 반복문을 이용해
거스름돈으로 나눔&나머지를 저장하는 방법으로 간단하게 풀 수 있었다.
2차원 배열에 메모리 할당하는 법
int i, n = 10;
int **p;
p = (int**)malloc(sizeof(int*)*n);
for(i=0; i<10; i++) p[i] = (int*)malloc(sizeof(int)*n);
반복문을 이용해 할당한다.
메모리할당없이 푼 코드
#include <stdio.h>
int main(void) {
int test_case, T;
scanf("%d", &T);
for (test_case = 1; test_case <= T; test_case++) {
int money[8] = { 50000,10000,5000,1000,500,100,50,10 };
int cnt[8] = { 0 };
int N;
scanf("%d", &N);
for (int i = 0; i < 8; i++) {
cnt[i] = N / money[i];
N %= money[i];
}
printf("#%d\n", test_case);
for (int i = 0; i < 8; i++) {
printf("%d ", cnt[i]);
}
printf("\n");
}
return 0;
}
방법은 같다. 거스름돈을 배열에 저장 후 거스름돈의 개수를 저장할 배열에 N을 거스름돈으로 나눈 몫을 저장하면 된다.
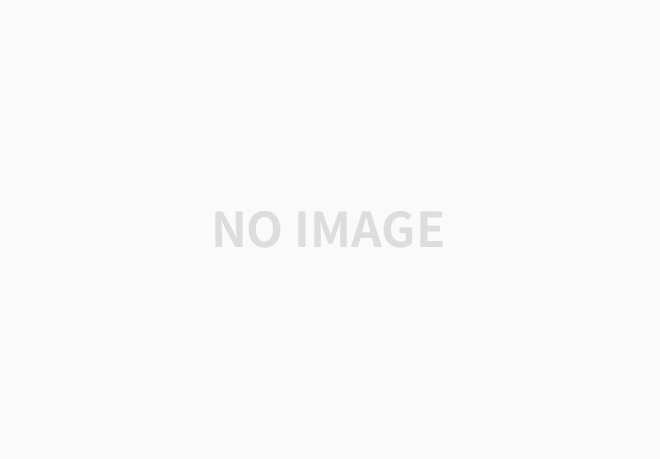
'SWEA > [D2]' 카테고리의 다른 글
[C언어] SWEA 1204 최빈수 구하기 (0) | 2020.08.15 |
---|---|
[C언어] SWEA 1284 수도요금경쟁 (0) | 2020.08.15 |
[C언어] SWEA 1989 초심자의 회문검사 (0) | 2020.08.09 |
[C언어] SWEA 1926 간단한 369게임 (0) | 2020.07.19 |
[C언어] SWEA 1945 간단한 소인수분해 (0) | 2020.07.17 |